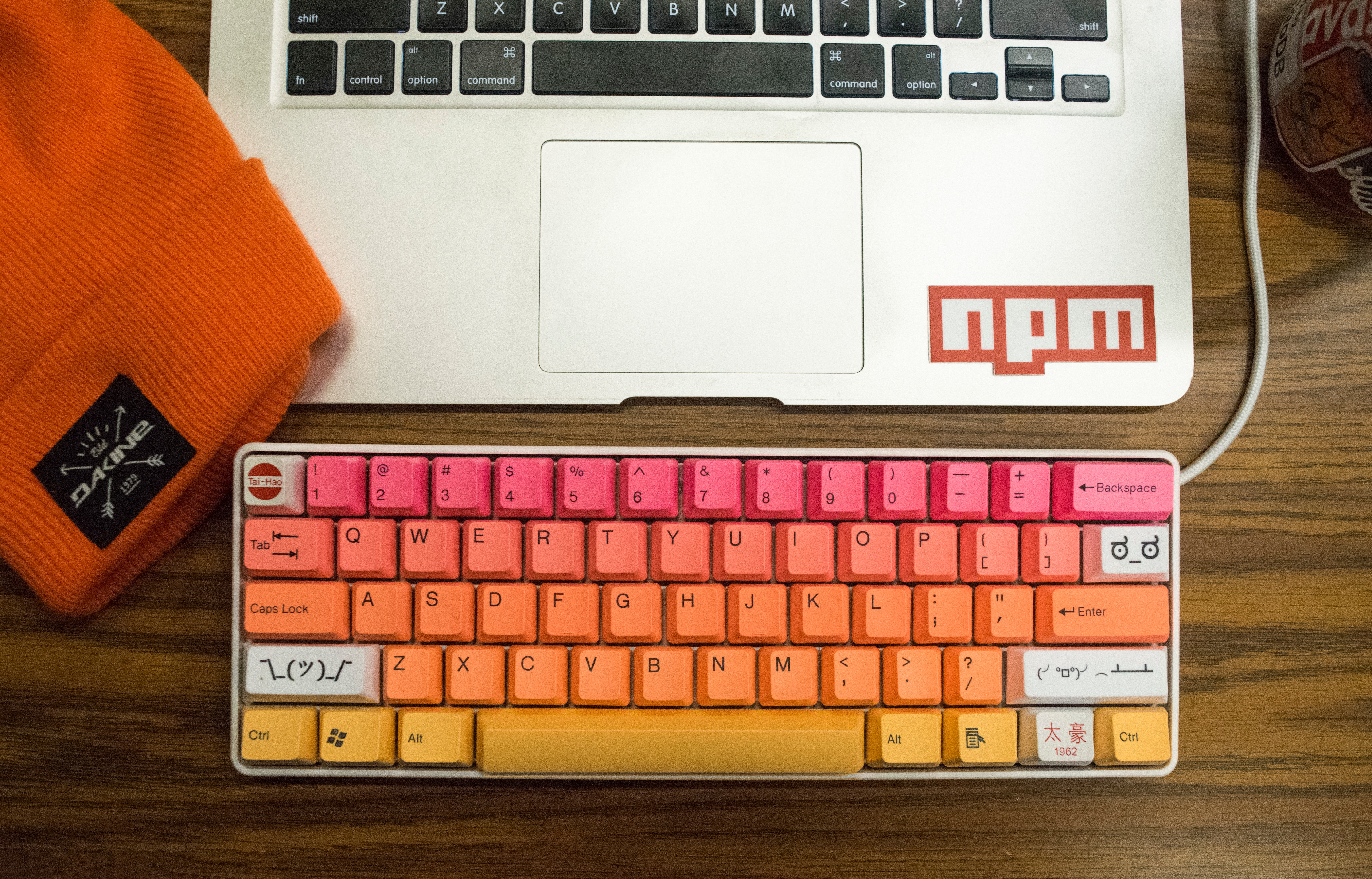
Javascript 如何替换所有字符串
在 Javascript 中如何替换字符串,这个题目在 stack overflow 竟然拥有 4 百多万的阅读量。所以说基础的东西才是最多人需要的。因此,今天我们就来深入理解一下,如何在 Javascript 中替换所有的字符串。
在 JavaScript 中,我们可以使用字符串的 replace()方法来替换字符串。但是,这个方法只会替换第一个匹配项,如果我们想要替换所有匹配项,需要在正则表达式中使用 g 标志(全局匹配)。
下面是一个示例代码,展示如何替换所有匹配项:
let str = "This is a test string. This is another test string.";
let newStr = str.replace(/test/g, "example");
console.log(newStr);
// 输出:This is a example string. This is another example string.
在上面的示例中,我们将所有的"test"字符串替换成"example"字符串。使用正则表达式时,我们将 g 标志添加到正则表达式的末尾,表示全局匹配。这样,replace()方法就会将所有匹配项都替换掉。
需要注意的是,replace()方法不会更改原始字符串,而是返回一个新的字符串。如果要更改原始字符串,可以将新字符串赋值回原始字符串,或者直接在原始字符串上进行操作。
除了使用正则表达式,我们还可以使用第三方库如 Lodash 或 Underscore 来进行字符串替换。这些库提供了更丰富的字符串处理功能,可以大大简化我们的编程工作。例如,Lodash 提供了 replace()方法的替代品 replace()和 replaceAll(),可以方便地替换所有匹配项。
// 使用Lodash替换所有匹配项
let newStr = _.replace(str, /test/g, "example");
let newStr2 = _.replaceAll(str, "test", "example");
console.log(newStr);
// 输出:This is a example string. This is another example string.
console.log(newStr2);
// 输出:This is a example string. This is another example string.
无论使用哪种方法,我们都可以轻松地在 JavaScript 中替换所有字符串。这个功能在许多应用程序中都非常有用,例如文本编辑器、搜索引擎等等。
需要注意的是,在使用正则表达式进行全局替换时,我们还需要小心一些细节。例如,如果我们在替换字符串中使用了$符号,可能会与正则表达式的替换模式发生冲突。在这种情况下,我们需要使用反斜杠来转义$符号,以避免产生意外的结果。
下面是一个示例代码,展示了在替换字符串中使用$符号时的注意事项:
let str = "This is a test string. This is another test string.";
let newStr = str.replace(/test/g, "$example");
console.log(newStr);
// 输出:This is a $example string. This is another $example string.
在上面的示例中,我们尝试将所有的"test"字符串替换成"$example"字符串。但是,由于$符号被视为替换模式,因此替换结果并不是我们所期望的。为了解决这个问题,我们需要使用反斜杠来转义$符号,如下所示:
let newStr = str.replace(/test/g, "\\$example");
console.log(newStr);
// 输出:This is a $example string. This is another $example string.
在上面的代码中,我们使用了双反斜杠来转义$符号,这样就能正确地替换所有匹配项了。
在实际应用中,我们可能需要根据具体情况对正则表达式进行调整,以实现更精确的匹配和替换。但无论如何,掌握字符串的替换方法是 Web 开发中非常重要的一步,它可以帮助我们轻松地处理各种文本数据,从而构建出更加强大和灵活的应用程序。
2021 年,String.replaceAll()
方法已经写进 ECMAScript 2021 language specification。可以点击这里查看。
所以,最简单的方法是使用 Javascript 的replaceAll
。
const p =
"The quick brown fox jumps over the lazy dog. If the dog reacted, was it really lazy?";
console.log(p.replaceAll("dog", "monkey"));
// Expected output: "The quick brown fox jumps over the lazy monkey. If the monkey reacted, was it really lazy?"
// Global flag required when calling replaceAll with regex
const regex = /Dog/gi;
console.log(p.replaceAll(regex, "ferret"));
// Expected output: "The quick brown fox jumps over the lazy ferret. If the ferret reacted, was it really lazy?"